Introduction to atomman: Gamma surface plotting
Lucas M. Hale, lucas.hale@nist.gov, Materials Science and Engineering Division, NIST.
1. Introduction
This Notebook outlines the GammaSurface class and the various stacking fault plots that it can generate. See the 4.3. Stacking fault generator Notebook for generating systems containing stacking faults.
Library Imports
[1]:
# Standard Python libraries
from pathlib import Path
import datetime
# http://www.numpy.org/
import numpy as np
# https://github.com/usnistgov/atomman
import atomman as am
import atomman.unitconvert as uc
# https://matplotlib.org/
import matplotlib.pyplot as plt
%matplotlib inline
# Show atomman version
print('atomman version =', am.__version__)
# Show date of Notebook execution
print('Notebook executed on', datetime.date.today())
atomman version = 1.4.11
Notebook executed on 2024-04-29
2. Underlying theory
Generalized stacking fault energy maps (\(\gamma\)-surfaces) provide 2D maps of the stacking fault energy associated with arbitrary shifts along a crystallographic fault plane. Typically, the fault plane corresponds to one of the crystal’s slip planes and the stacking fault energies and pathways influence dislocation structures and dislocation slip.
A \(\gamma\)-surface is defined by the crystal and the fault plane. Starting in an unfaulted configuration, the atoms on one side of the fault plane are shifted by a vector \(\mathbf{d}\) which is within the fault plane. The generalized stacking fault energy for a given shift, \(\gamma_{gsf}(\mathbf{d})\), is computed as the difference in potential energy, \(U\) of the shifted configuration relative to the corresponding unshifted configuration (\(\mathbf{d}=\mathbf{0}\)) divided by the fault plane area, \(A_{fault}\)
The measured \(\gamma_{gsf}\) values may be “unrelaxed”, in which case no atomic relaxations are allowed, or “relaxed”, in which atoms are allowed to move only in the direction normal to the slip plane to lower the system’s energy. For relaxed \(\gamma\)-surfaces, the relaxation distance, \(\delta_{\bot}\), can be evaluated using the centers of mass of the atoms on the two sides of the fault plane, \(\mathbf{R}^+\) and \(\mathbf{R}^-\), and measuring how the plane normal component \(\bot\) changes relative to the unshifted state
As a \(\gamma\)-surface is a 2D map, the applied shift, \(\mathbf{d}\), can be expressed using two non-parallel vectors within the fault plane, \(\mathbf{a_1}\) and \(\mathbf{a_2}\). Taking \(\mathbf{a_1}\) and \(\mathbf{a_2}\) as lattice vectors (i.e. vectors between two symetrically identical lattice sites) will define a 2D cell encompassing all possible unique stacking fault shifts for the fault plane. \(\mathbf{d}\) can then be represented using fractional coordinates, \(a_1\) and \(a_2\), relative to \(\mathbf{a_1}\) and \(\mathbf{a_2}\)
The \(\gamma_{gsf}\) (and \(\delta_{\bot}\)) data is fit to interpolation models to provide continuous values between the measured points. Two interpolation models are fit to the data
A multiquadric radial basis function interpolation.
A nearest-value interpolation.
To perform the fits, the data is replicated across the periodic bounds by shifting the \(a_1\) and \(a_2\) values by \(\pm 1\). The unique \(a_1\) and \(a_2\) values less than and closest to 0 and greater than and closest to 1 are identified, and all measured points between these two limits are fed into the fitting models to ensure smooth representation across the bounds. Any arbitrary shift values \(\mathbf{d}\) are converted into \(a_1\) and \(a_2\) values between 0 and 1 for use in retrieving values from the fitted models.
3. Defining gamma surface data
The atomman.defect.GammaSurface class offers interpolation and plotting options for generalized stacking fault data.
3.1. Setting values
The stacking fault data can be set directly either during class initialization or by using the GammaSurface.set() method.
Parameters:
a1vect (array-like object of length 3) The a1 shifting vector. If box is given, a1vect is taken as a crystal lattice vector, otherwise as a Cartesian vector.
a2vect (array-like object of length 3) The a2 shifting vector. If box is given, a1vect is taken as a crystal lattice vector, otherwise as a Cartesian vector.
a1 (array-like object of length N) List of fractional coordinates along a1vect corresponding to the E_gsf (and delta) values.
a2 (array-like object of length N) List of fractional coordinates along a2vect corresponding to the E_gsf (and delta) values.
E_gsf (array-like object of length N) List of generalized stacking fault energies for the positions associated with the corresponding (a1, a2) fractional coordinates.
box (atomman.Box, optional) Defines unit cell box dimensions for conversion between crystal lattice and Cartesian vectors. If not given, will be set as a square unit box, thus no conversion will occur (i.e. a1vect, a2vect will be Cartesian).
delta (array-like object of length N, optional) List of change in displacements normal to the fault plane for the positions associated with the corresponding (a1, a2) fractional coordinates.
[2]:
# Parameters for an Al fcc 111 stacking fault using 2008--Mendelev-M-I--Al--LAMMPS--ipr1
box = am.Box.cubic(a=4.045259794316263)
a1vect = np.array([ 0.0, -0.5, 0.5])
a2vect = np.array([ 0.5, -0.5, 0.0])
# Measured 30x30 grid of generalized stacking fault data
a1 = np.array([0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667, 0.0, 0.03333333333333333, 0.06666666666666667, 0.1, 0.13333333333333333, 0.16666666666666666, 0.2, 0.23333333333333334, 0.26666666666666666, 0.3, 0.3333333333333333, 0.36666666666666664, 0.4, 0.43333333333333335, 0.4666666666666667, 0.5, 0.5333333333333333, 0.5666666666666667, 0.6, 0.6333333333333333, 0.6666666666666666, 0.7, 0.7333333333333333, 0.7666666666666666, 0.8, 0.8333333333333334, 0.8666666666666667, 0.9, 0.9333333333333333, 0.9666666666666667])
a2 = np.array([0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.03333333333333333, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.06666666666666667, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.1, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.13333333333333333, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.16666666666666666, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.2, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.23333333333333334, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.26666666666666666, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.3333333333333333, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.36666666666666664, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.4, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.43333333333333335, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.4666666666666667, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5333333333333333, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.5666666666666667, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6333333333333333, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.6666666666666666, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7333333333333333, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.7666666666666666, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8333333333333334, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.8666666666666667, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9333333333333333, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667, 0.9666666666666667])
E_gsf = np.array([0.0, 0.0003276644660250489, 0.0013197032610667036, 0.0030080286990708206, 0.005299272889367739, 0.007853645516566367, 0.010344679818558007, 0.012746832373238334, 0.015027073432287454, 0.01713303089875586, 0.018964037324507355, 0.020359502522962633, 0.021289405537333824, 0.021852691822297415, 0.022145827289685307, 0.022235037181376597, 0.022145827289685307, 0.021852691822297415, 0.021289405537333824, 0.020359502522962633, 0.018964037324507355, 0.01713303089875586, 0.015027073432287454, 0.012746832373238334, 0.010344679818558007, 0.007853645516566367, 0.005299272889367739, 0.0030080286990708206, 0.0013197032610667036, 0.0003276644660250489, 0.0003276644660250489, 0.0009624286188679017, 0.0022516168853462543, 0.004179318519958506, 0.006502012537909789, 0.008836360637079085, 0.011071402674020946, 0.013163772621198347, 0.015069987998274524, 0.01676775797378991, 0.018202365560353467, 0.019266421370540104, 0.01992937325132262, 0.02028149454107946, 0.02042805135939521, 0.02042805137633013, 0.02028149454107946, 0.01992937325132262, 0.019266421370540104, 0.018202365560353467, 0.01676775797378991, 0.015069987998274524, 0.013163772621198347, 0.011071402674020946, 0.008836360637079085, 0.006502012537909789, 0.004179318519958506, 0.0022516168853462543, 0.0009624286188679017, 0.0003276644660250489, 0.0013197032610667036, 0.0022516168853462543, 0.00379342830708924, 0.005803543732471961, 0.00793645979564411, 0.010002224995159091, 0.011913305022139525, 0.013596628277462183, 0.015034223959459074, 0.016248082833591557, 0.017231759917201616, 0.017942818177434202, 0.01838247984870989, 0.018588416437931753, 0.018643586130194265, 0.018588416437931753, 0.01838247984870989, 0.017942818177434202, 0.017231759917201616, 0.016248082833591557, 0.015034223959459074, 0.013596628277462183, 0.011913305022139525, 0.010002224995159091, 0.00793645979564411, 0.005803543732471961, 0.00379342830708924, 0.0022516168853462543, 0.0013197032610667036, 0.001008906143675133, 0.0030080286990708206, 0.004179318519958506, 0.005803543732471961, 0.007637852288274728, 0.009496660797274951, 0.011243532636952849, 0.012743928196872886, 0.013936123292691541, 0.014847319113605083, 0.015549856853502065, 0.016082846740739055, 0.01645862282198508, 0.016705110082744275, 0.01682916112649225, 0.01682916112649225, 0.016705110082744275, 0.016458622823396964, 0.016082846740739055, 0.015549856853502065, 0.014847319113605083, 0.013936123292691541, 0.012743928195463569, 0.011243532636952849, 0.009496660797274951, 0.007637852288274728, 0.005803543732471961, 0.004179318519958506, 0.0030080286990708206, 0.0023998946753737717, 0.0023998946753737717, 0.005299272889367739, 0.006502012537909789, 0.00793645979564411, 0.009496660797274951, 0.011035628677366563, 0.01237392710762056, 0.013385589574354801, 0.01404488393495149, 0.014423942718867563, 0.014639725692955295, 0.014765683854799951, 0.014838711638586797, 0.014883062600264814, 0.01489480692722371, 0.014883062600264814, 0.014838711638586797, 0.014765683854799951, 0.014639725692955295, 0.014423942718867563, 0.01404488393495149, 0.013385589574354801, 0.01237392710762056, 0.011035628677366563, 0.009496660797274951, 0.00793645979564411, 0.006502012537909789, 0.005299272889367739, 0.004494424933099573, 0.004211052927859079, 0.004494424933099573, 0.007853645516566367, 0.008836360637079085, 0.010002224995159091, 0.011243532636952849, 0.01237392710762056, 0.013222604888781, 0.013701533360467543, 0.013831808215880651, 0.013724810143582959, 0.01351871945764962, 0.013288096454131046, 0.013053141110424394, 0.01288473799574951, 0.01288473799574951, 0.013053141110424394, 0.013288096454131046, 0.01351871945764962, 0.013724810143582959, 0.013831808215880651, 0.013701533360467543, 0.013222604888781, 0.01237392710762056, 0.011243532636952849, 0.010002224995159091, 0.008836360637079085, 0.007853645516566367, 0.007090113076005102, 0.006654577493741669, 0.006654577493741669, 0.007090113076005102, 0.010344679818558007, 0.011071402674020946, 0.011913305022139525, 0.012743928196872886, 0.013385589574354801, 0.013701533360467543, 0.013650790504842861, 0.013297636384243845, 0.012775745469630308, 0.01220434858125911, 0.011633781497279536, 0.011198475775133013, 0.011035966519916687, 0.011198475775133013, 0.011633781497279536, 0.01220434858125911, 0.012775745469630308, 0.013297636384243845, 0.013650790504842861, 0.013701533360467543, 0.013385589574354801, 0.012743928195463569, 0.011913305022139525, 0.011071402674020946, 0.010344679818558007, 0.009791920945171492, 0.009439135467834685, 0.009310068611476237, 0.009439135467834685, 0.009791920945171492, 0.012746832373238334, 0.013163772621198347, 0.013596628277462183, 0.013936123292691541, 0.01404488393495149, 0.013831808215880651, 0.013297636384243845, 0.012529422359386078, 0.011638354833823921, 0.01074148627431762, 0.010038128724558996, 0.009656642364574372, 0.009656642364574372, 0.010038128724558996, 0.01074148627431762, 0.011638354833823921, 0.012529422359386078, 0.013297636384243845, 0.013831808215880651, 0.01404488393495149, 0.013936123292691541, 0.013596628277462183, 0.013163772621198347, 0.012746832373238334, 0.012404093212327083, 0.012183307197516747, 0.012090801183497172, 0.012090801183497172, 0.012183307197516747, 0.012404093212327083, 0.015027073432287454, 0.015069987998274524, 0.015034223959459074, 0.014847319113605083, 0.014423942718867563, 0.013724810143582959, 0.012775745469630308, 0.011638354833823921, 0.010430230660255049, 0.009449322004070611, 0.008844617563989746, 0.00864189871015902, 0.008844617563989746, 0.009449322004070611, 0.010430230660255049, 0.011638354833823921, 0.012775745469630308, 0.013724810143582959, 0.014423942718867563, 0.014847319113605083, 0.015034223959459074, 0.015069987998274524, 0.015027073432287454, 0.014934876376596327, 0.01483061319175465, 0.014740550396192733, 0.014701162449128703, 0.014740550396192733, 0.01483061319175465, 0.014934876376596327, 0.01713303089875586, 0.01676775797378991, 0.016248082833591557, 0.015549856853502065, 0.014639725692955295, 0.01351871945764962, 0.01220434858125911, 0.01074148627431762, 0.009449322004070611, 0.008578811099234605, 0.00815484933711049, 0.00815484933711049, 0.008578811099234605, 0.009449322004070611, 0.01074148627431762, 0.01220434858125911, 0.01351871945764962, 0.014639725692955295, 0.015549856853502065, 0.016248082833591557, 0.01676775797378991, 0.01713303089875586, 0.01732800024220794, 0.017369215082978437, 0.017321437948693633, 0.017272284193973794, 0.017272284193973794, 0.017321437948693633, 0.017369215082978437, 0.01732800024220794, 0.018964037324507355, 0.018202365560353467, 0.017231759917201616, 0.016082846740739055, 0.014765683854799951, 0.013288096454131046, 0.011633781497279536, 0.010038128724558996, 0.008844617563989746, 0.00815484933711049, 0.007931982282131142, 0.00815484933711049, 0.008844617563989746, 0.010038128724558996, 0.011633781497279536, 0.013288096454131046, 0.014765683854799951, 0.016082846740739055, 0.017231759917201616, 0.018202365560353467, 0.018964037324507355, 0.019454139208464893, 0.019694360589358625, 0.01977827285566793, 0.01978984714716384, 0.019786649819980306, 0.01978984714716384, 0.01977827285566793, 0.019694360589358625, 0.019454139208464893, 0.020359502522962633, 0.019266421370540104, 0.017942818177434202, 0.01645862282198508, 0.014838711638586797, 0.013053141110424394, 0.011198475775133013, 0.009656642364574372, 0.00864189871015902, 0.00815484933711049, 0.00815484933711049, 0.00864189871015902, 0.009656642364574372, 0.011198475775133013, 0.013053141110424394, 0.014838711638586797, 0.016458622823396964, 0.017942818177434202, 0.019266421370540104, 0.020359502522962633, 0.021147064770108075, 0.021649358382385377, 0.021934945102187603, 0.022076143474118026, 0.022128736159221365, 0.022128736159221365, 0.022076143474118026, 0.021934945102187603, 0.021649358382385377, 0.021147064770108075, 0.021289405537333824, 0.01992937325132262, 0.01838247984870989, 0.016705110082744275, 0.014883062600264814, 0.01288473799574951, 0.011035966519916687, 0.009656642364574372, 0.008844617563989746, 0.008578811099234605, 0.008844617563989746, 0.009656642364574372, 0.011035966519916687, 0.01288473799574951, 0.014883062600264814, 0.016705110082744275, 0.01838247984870989, 0.01992937325132262, 0.021289405537333824, 0.022370099121114623, 0.0231503150713325, 0.02366759427728574, 0.023980226181548533, 0.024139292094786883, 0.02418571534118722, 0.024139292094786883, 0.023980226181548533, 0.02366759427728574, 0.0231503150713325, 0.022370099121114623, 0.021852691822297415, 0.02028149454107946, 0.018588416437931753, 0.01682916112649225, 0.01489480692722371, 0.01288473799574951, 0.011198475775133013, 0.010038128724558996, 0.009449322004070611, 0.009449322004070611, 0.010038128724558996, 0.011198475775133013, 0.01288473799574951, 0.01489480692722371, 0.01682916112649225, 0.018588416437931753, 0.02028149454107946, 0.021852691822297415, 0.02317845769263327, 0.024204824269097428, 0.024942382102965746, 0.025433018993558404, 0.02572289378519483, 0.02585156812403108, 0.02585156812403108, 0.02572289378519483, 0.025433018993558404, 0.024942382102965746, 0.024204824284620466, 0.02317845769263327, 0.022145827289685307, 0.02042805135939521, 0.018643586130194265, 0.01682916112649225, 0.014883062600264814, 0.013053141110424394, 0.011633781497279536, 0.01074148627431762, 0.010430230660255049, 0.01074148627431762, 0.011633781497279536, 0.013053141110424394, 0.014883062600264814, 0.01682916112649225, 0.018643586130194265, 0.02042805135939521, 0.022145827289685307, 0.023657583085668364, 0.02488053126382751, 0.025800253053947248, 0.02644560104894149, 0.026860034602867854, 0.02708309171483324, 0.027151657016416905, 0.02708309171483324, 0.026860034602867854, 0.026445601050353373, 0.025800253069470282, 0.02488053126382751, 0.023657583085668364, 0.022235037182788485, 0.02042805135939521, 0.018588416437931753, 0.016705110082744275, 0.014838711638586797, 0.013288096454131046, 0.01220434858125911, 0.011638354833823921, 0.011638354833823921, 0.01220434858125911, 0.013288096454131046, 0.014838711638586797, 0.016705110082744275, 0.018588416437931753, 0.02042805135939521, 0.022235037181376597, 0.023876272100392736, 0.02525037551288545, 0.02631760912831916, 0.027088755361362748, 0.027603533296746703, 0.027908712191780646, 0.028046898021049052, 0.028046898021049052, 0.027908712191780646, 0.027603533296746703, 0.027088755351484688, 0.02631760912831916, 0.02525037551288545, 0.023876272100392736, 0.022145827289685307, 0.02028149454107946, 0.01838247985011921, 0.016458622823396964, 0.014765683854799951, 0.01351871945764962, 0.012775745469630308, 0.012529422359386078, 0.012775745469630308, 0.01351871945764962, 0.014765683854799951, 0.016458622823396964, 0.01838247984870989, 0.02028149454107946, 0.022145827289685307, 0.023876272100392736, 0.02536675249494844, 0.02655738371046003, 0.027439093842301504, 0.028041035722972417, 0.02841200786241034, 0.028605543432288737, 0.028664313998241875, 0.028605543432288737, 0.02841200786241034, 0.028041035722972417, 0.027439093842301504, 0.026557383707638826, 0.02536675249494844, 0.02387627209898085, 0.021852691822297415, 0.01992937325132262, 0.017942818177434202, 0.016082846740739055, 0.014639725692955295, 0.013724810143582959, 0.013297636384243845, 0.013297636384243845, 0.013724810143582959, 0.014639725692955295, 0.016082846740739055, 0.017942818177434202, 0.01992937325132262, 0.021852691822297415, 0.023657583085668364, 0.02525037551288545, 0.026557383711871916, 0.02754913586481439, 0.02824061569970574, 0.028677500498590372, 0.02892070902513713, 0.029025523592854756, 0.029025523585797897, 0.02892070902513713, 0.028677500494354715, 0.02824061569970574, 0.027549135866226274, 0.026557383707638826, 0.02525037551288545, 0.023657583085668364, 0.021289405537333824, 0.019266421370540104, 0.017231759917201616, 0.015549856853502065, 0.014423942718867563, 0.013831808215880651, 0.013650790504842861, 0.013831808215880651, 0.014423942718867563, 0.015549856853502065, 0.017231759917201616, 0.019266421370540104, 0.021289405537333824, 0.02317845769263327, 0.02488053126382751, 0.02631760912831916, 0.027439093839480302, 0.028240615698293857, 0.028759317463963645, 0.02905994257120264, 0.029210986008471558, 0.029256067117124782, 0.029210986008471558, 0.029059942572617094, 0.028759317463963645, 0.02824061569970574, 0.027439093840892187, 0.02631760912831916, 0.02488053126382751, 0.02317845769263327, 0.020359502522962633, 0.018202365560353467, 0.016248082833591557, 0.014847319113605083, 0.01404488393495149, 0.013701533360467543, 0.013701533360467543, 0.01404488393495149, 0.014847319113605083, 0.016248082833591557, 0.018202365560353467, 0.020359502522962633, 0.022370099121114623, 0.024204824269097428, 0.02580025305253536, 0.027088755361362748, 0.028041035722972417, 0.028677500488709744, 0.02905994257402641, 0.029267261304526674, 0.029356079089374668, 0.029356079072439745, 0.02926726130593856, 0.029059942572617094, 0.02867750049012163, 0.028041035722972417, 0.027088755361362748, 0.02580025305253536, 0.024204824269097428, 0.022370099121114623, 0.018964037324507355, 0.01676775797378991, 0.015034223959459074, 0.013936123292691541, 0.013385589574354801, 0.013222604888781, 0.013385589574354801, 0.013936123292691541, 0.015034223959459074, 0.01676775797378991, 0.018964037324507355, 0.021147064770108075, 0.0231503150713325, 0.024942382102965746, 0.02644560104894149, 0.027603533296746703, 0.02841200786241034, 0.02892070902513713, 0.029210986008471558, 0.02935607908796278, 0.02939912316653883, 0.029356079090783985, 0.029210986008471558, 0.02892070902513713, 0.02841200786241034, 0.027603533296746703, 0.02644560104894149, 0.024942382102965746, 0.0231503150713325, 0.021147064770108075, 0.01713303089875586, 0.015069987998274524, 0.013596628277462183, 0.012743928196872886, 0.01237392710762056, 0.01237392710762056, 0.012743928196872886, 0.013596628277462183, 0.015069987998274524, 0.01713303089875586, 0.019454139208464893, 0.021649358382385377, 0.02366759427728574, 0.025433018993558404, 0.026860034602867854, 0.027908712191780646, 0.028605543432288737, 0.029025523585797897, 0.029256067143937765, 0.029356079089374668, 0.029356079086553463, 0.029256067143937765, 0.029025523584386013, 0.028605543432288737, 0.027908712191780646, 0.02686003460710351, 0.025433018993558404, 0.02366759427728574, 0.021649358382385377, 0.019454139208464893, 0.015027073432287454, 0.013163772621198347, 0.011913305022139525, 0.011243532635540964, 0.011035628677366563, 0.011243532636952849, 0.011913305022139525, 0.013163772621198347, 0.015027073432287454, 0.01732800024220794, 0.019694360589358625, 0.021934945102187603, 0.023980226181548533, 0.02572289378519483, 0.02708309171483324, 0.028046898021049052, 0.028664313998241875, 0.02902552359567596, 0.029210986008471558, 0.02926726130593856, 0.029210986008471558, 0.029025523584386013, 0.028664313998241875, 0.028046898021049052, 0.02708309171483324, 0.02572289378519483, 0.023980226181548533, 0.021934945102187603, 0.019694360589358625, 0.01732800024220794, 0.012746832373238334, 0.011071402674020946, 0.010002224995159091, 0.009496660797274951, 0.009496660797274951, 0.010002224995159091, 0.011071402674020946, 0.012746832373238334, 0.014934876376596327, 0.017369215082978437, 0.01977827285566793, 0.022076143474118026, 0.024139292094786883, 0.02585156812403108, 0.027151657016416905, 0.028046898021049052, 0.028605543432288737, 0.02892070902513713, 0.02905994257402641, 0.02905994257120264, 0.02892070902513713, 0.028605543432288737, 0.028046898021049052, 0.027151657016416905, 0.02585156812403108, 0.024139292094786883, 0.022076143474118026, 0.01977827285566793, 0.017369215082978437, 0.014934876376596327, 0.010344679818558007, 0.008836360637079085, 0.00793645979564411, 0.007637852288274728, 0.00793645979564411, 0.008836360637079085, 0.010344679818558007, 0.012404093212327083, 0.01483061319175465, 0.017321437948693633, 0.01978984714716384, 0.022128736159221365, 0.02418571534118722, 0.02585156812403108, 0.02708309171483324, 0.027908712191780646, 0.02841200786241034, 0.028677500494354715, 0.028759317463963645, 0.028677500502820894, 0.02841200786241034, 0.027908712191780646, 0.02708309171483324, 0.02585156812403108, 0.02418571534118722, 0.022128736159221365, 0.01978984714716384, 0.017321437948693633, 0.01483061319175465, 0.012404093212327083, 0.007853645516566367, 0.006502012537909789, 0.005803543732471961, 0.005803543732471961, 0.006502012537909789, 0.007853645516566367, 0.009791920945171492, 0.012183307197516747, 0.014740550396192733, 0.017272284193973794, 0.019786649819980306, 0.022128736159221365, 0.024139292094786883, 0.02572289378519483, 0.026860034602867854, 0.027603533296746703, 0.028041035722972417, 0.028240615708174484, 0.028240615692648883, 0.028041035722972417, 0.027603533296746703, 0.026860034602867854, 0.02572289378519483, 0.024139292094786883, 0.022128736159221365, 0.019786649819980306, 0.017272284193973794, 0.014740550396192733, 0.012183307197516747, 0.009791920945171492, 0.005299272889367739, 0.004179318519958506, 0.00379342830708924, 0.004179318519958506, 0.005299272889367739, 0.007090113076005102, 0.009439135467834685, 0.012090801183497172, 0.014701162449128703, 0.017272284193973794, 0.01978984714716384, 0.022076143474118026, 0.023980226181548533, 0.025433018993558404, 0.02644560104894149, 0.027088755361362748, 0.027439093840892187, 0.027549135866226274, 0.027439093840892187, 0.027088755361362748, 0.026445601050353373, 0.025433018993558404, 0.023980226181548533, 0.022076143474118026, 0.01978984714716384, 0.017272284193973794, 0.014701162449128703, 0.012090801183497172, 0.009439135467834685, 0.007090113076005102, 0.0030080286990708206, 0.0022516168853462543, 0.0022516168853462543, 0.0030080286990708206, 0.004494424933099573, 0.006654577493741669, 0.009310068611476237, 0.012090801183497172, 0.014740550396192733, 0.017321437948693633, 0.01977827285566793, 0.021934945102187603, 0.02366759427728574, 0.024942382102965746, 0.02580025305253536, 0.02631760912831916, 0.026557383725983066, 0.02655738371046003, 0.02631760912831916, 0.025800253068058398, 0.024942382102965746, 0.02366759427728574, 0.021934945102187603, 0.01977827285566793, 0.017321437948693633, 0.014740550396192733, 0.012090801183497172, 0.009310068611476237, 0.006654577493741669, 0.004494424933099573, 0.0013197032610667036, 0.0009624286188679017, 0.0013197032610667036, 0.0023998946753737717, 0.004211052927859079, 0.006654577493741669, 0.009439135467834685, 0.012183307197516747, 0.01483061319175465, 0.017369215082978437, 0.019694360589358625, 0.021649358382385377, 0.0231503150713325, 0.024204824269097428, 0.02488053126382751, 0.02525037551288545, 0.02536675249494844, 0.02525037551288545, 0.02488053126382751, 0.024204824269097428, 0.0231503150713325, 0.021649358382385377, 0.019694360589358625, 0.017369215082978437, 0.01483061319175465, 0.012183307197516747, 0.009439135467834685, 0.006654577493741669, 0.004211052927859079, 0.0023998946753737717, 0.0003276644660250489, 0.0003276644660250489, 0.001008906143675133, 0.0023998946753737717, 0.004494424933099573, 0.007090113076005102, 0.009791920945171492, 0.012404093212327083, 0.014934876376596327, 0.01732800024220794, 0.019454139208464893, 0.021147064770108075, 0.022370099121114623, 0.02317845769263327, 0.023657583085668364, 0.023876272100392736, 0.023876272100392736, 0.023657583085668364, 0.02317845769263327, 0.022370099121114623, 0.021147064770108075, 0.019454139208464893, 0.01732800024220794, 0.014934876376596327, 0.012404093212327083, 0.009791920945171492, 0.007090113076005102, 0.004494424933099573, 0.0023998946753737717, 0.001008906143675133])
delta = np.array([0.0, 0.005541623615307856, 0.01943663001532059, 0.03815843253480722, 0.0606882120312946, 0.08851254231706207, 0.11262352593976033, 0.13075899586557682, 0.14592595011600196, 0.16081955557027783, 0.17970188538780008, 0.20295571167149262, 0.22237239074203075, 0.23533048963622605, 0.24255613160673306, 0.24485152496497165, 0.24255613160670464, 0.23533048963622605, 0.22237239074203075, 0.20295571167145, 0.1797018853878285, 0.16081955557027072, 0.14592595011597354, 0.13075899586556972, 0.11262352593979585, 0.08851254231706207, 0.06068821203162145, 0.038158432534842746, 0.019436630015356116, 0.005541623615314961, 0.005541623615314961, 0.01331536400725497, 0.026866349109880616, 0.04561181437118478, 0.07078901159886186, 0.097001190583768, 0.11689842506950754, 0.13270331901448884, 0.14591871007265667, 0.15834458933661466, 0.17380584828498513, 0.19338513562863824, 0.2120728816228592, 0.22341833236870912, 0.22853662554950915, 0.2285088882561297, 0.2234183323688157, 0.212072881622845, 0.1933851356287093, 0.17380584828498513, 0.15834458933660756, 0.1459187100726922, 0.13270331901449595, 0.11689842506949333, 0.097001190583768, 0.07078901159886186, 0.0456118143712132, 0.026866349109866405, 0.013315364007198127, 0.005541623615314961, 0.019436630015306378, 0.026866349109880616, 0.04005475255581814, 0.060689274713794816, 0.0854178570514108, 0.10542699280703971, 0.1221348536247433, 0.13608731348548986, 0.14743483944548075, 0.1576976308827085, 0.1697128415987521, 0.18368435752065437, 0.19836540958823434, 0.2090258538200942, 0.21233186398202974, 0.20902585382006578, 0.19836540958827698, 0.18368435752063306, 0.1697128415987592, 0.15769763088269428, 0.14743483944548075, 0.13608731348551828, 0.12213485362440224, 0.10542699280705392, 0.0854178570514108, 0.060689274713794816, 0.04005475255581814, 0.026866349109880616, 0.019436630015306378, 0.016933037985893407, 0.038158432534856956, 0.04561181437119899, 0.060689274713794816, 0.08120115836113939, 0.0985535759678342, 0.1136411208109962, 0.12704318624997768, 0.13834820487858224, 0.1472848113428853, 0.15479230638712238, 0.16284388405492933, 0.17061694797637017, 0.1780824270538659, 0.18454289853671924, 0.18454289853670502, 0.1780824270538659, 0.1706169479749704, 0.1628438840549009, 0.15479230638761976, 0.14728481134284976, 0.13834820487859645, 0.12704318624994215, 0.1136411208109962, 0.0985535759678342, 0.08120115836112518, 0.060689274713794816, 0.045611814371206094, 0.038158432534856956, 0.03496863398257943, 0.03496863398256522, 0.06068821203132302, 0.07078901159886186, 0.0854178570514108, 0.0985535759678342, 0.11024942418015371, 0.1209387863482192, 0.13037694682464007, 0.13781003362569777, 0.1430205577629522, 0.14663063092552875, 0.14968567222995688, 0.1508396907782128, 0.14868200566931478, 0.14648675448955828, 0.14868200566931478, 0.1508396907782128, 0.1496856722299995, 0.14663063092551454, 0.1430205577629522, 0.13781003362566935, 0.13037694682461165, 0.1209387863482192, 0.1102494241801466, 0.09855357596785552, 0.0854178570514108, 0.07078901159886186, 0.060688212031408284, 0.05596552064464788, 0.05455642334713673, 0.05596552064464788, 0.08851254231704786, 0.0970011905837822, 0.10542699280705392, 0.11364112081096778, 0.1209387863482192, 0.12704355822202018, 0.13150759263152167, 0.13377651899799758, 0.13351709855599836, 0.13146397985184421, 0.12804739193582293, 0.12174453412077924, 0.11797663476669129, 0.1179766347666984, 0.12174453412078634, 0.12804739193582293, 0.13146397985184421, 0.13351709855594152, 0.13377651899798337, 0.13150759263147904, 0.1270435582220628, 0.1209387863482192, 0.1136411208109962, 0.1054269928070255, 0.0970011905837822, 0.08851254231706918, 0.08036626481417386, 0.07604824987025438, 0.07604824987024728, 0.08036626481417386, 0.11262352593978164, 0.11689842506949333, 0.1221348536243454, 0.12704318624995636, 0.13037694682461165, 0.13150759263147904, 0.12999248242272898, 0.12562595829174938, 0.11823345924230466, 0.10802295303169274, 0.09968008144792861, 0.09656462253290954, 0.09594391333588703, 0.09656462253290954, 0.09968008144792861, 0.10802295303169274, 0.11823345924230466, 0.1256259582917636, 0.12999248242270056, 0.1315075926315643, 0.13037694682456902, 0.12704318624994926, 0.12213485362481435, 0.11689842506950754, 0.11262352593979585, 0.10818940005012223, 0.10241054554438733, 0.09954551522132249, 0.1024105455442168, 0.10818940005012223, 0.13075899586557682, 0.13270331901449595, 0.13608731348548986, 0.13834820487858224, 0.13781003362571198, 0.1337765189980047, 0.12562595829174228, 0.11331183964553304, 0.0963336603516467, 0.08305319680786738, 0.0782538069812162, 0.07715372057158731, 0.07715372057154468, 0.07825380698126594, 0.08305319680786738, 0.0963336603516467, 0.11331183964554015, 0.1256259582917778, 0.13377651899797627, 0.13781003362571198, 0.13834820487859645, 0.13608731348547565, 0.13270331901448884, 0.1307589958655342, 0.12996798226794226, 0.1298695679650237, 0.13017196439994194, 0.13017196439994194, 0.1298695679650521, 0.12996798226791384, 0.14592595011597354, 0.14591871007267798, 0.14743483944548785, 0.1472848113428853, 0.1430205577629522, 0.1335170985559131, 0.11823345924230466, 0.0963336603516467, 0.07673142302581226, 0.06662538303910992, 0.06274130654364285, 0.061801329041863085, 0.06274130654362153, 0.06662538303911703, 0.0767314230258549, 0.0963336603516467, 0.11823345924230466, 0.1335170985559131, 0.1430205577629593, 0.1472848113428853, 0.14743483944548075, 0.14591871007267798, 0.14592595011597354, 0.14803652963334457, 0.1520827241579994, 0.15680287801059478, 0.15862805959559978, 0.15680287801059478, 0.1520827241580065, 0.14803652963333036, 0.16081955557027072, 0.15834458933662887, 0.1576976308827227, 0.1547923063876837, 0.14663063092550033, 0.13146397985184421, 0.10802295303169274, 0.08305319680786738, 0.06662538303913834, 0.05714791668831509, 0.05273872428253412, 0.05273872428253412, 0.057147916688293776, 0.06662538303912413, 0.08305319680786738, 0.10802295303169274, 0.13146397985184421, 0.14663063092550743, 0.15479230638744923, 0.1576976308827227, 0.15834458933660756, 0.1608195555702494, 0.1660858553276796, 0.1727832363621502, 0.1773777556823788, 0.17955776385486644, 0.17955776385476696, 0.17737775568241432, 0.17278323636013226, 0.16608585532771514, 0.1797018853878143, 0.17380584828499934, 0.1697128415987308, 0.16284388405490802, 0.14968567222996398, 0.12804739193582293, 0.09968008144792861, 0.07825380698125883, 0.06274130654362864, 0.052738724282562544, 0.04908088528014787, 0.052738724282527016, 0.06274130654362864, 0.07825380698135831, 0.09968008144792861, 0.12804739193582293, 0.1496856722299711, 0.16284388405492223, 0.1697128415987308, 0.17380584828497803, 0.17970188538778586, 0.187787002826461, 0.1938092073899753, 0.19748976356095227, 0.19966471494916505, 0.20053351038428957, 0.19966471494916505, 0.19748976356096648, 0.19380920738998242, 0.18778700282643257, 0.20295571167143578, 0.19338513562851034, 0.18368435752066148, 0.17061694797610727, 0.1508396907782199, 0.12174453412079345, 0.09656462253289533, 0.07715372057161574, 0.06180132904185598, 0.05273872428253412, 0.052738724282527016, 0.06180132904185598, 0.07715372057162284, 0.0965646225328669, 0.12174453412085029, 0.1508396907782128, 0.17061694797490645, 0.18368435752060464, 0.19338513562864534, 0.20295571167145, 0.21056922427537472, 0.21580737042108922, 0.21943396764223166, 0.22183205361282887, 0.22334916339339372, 0.22334916339340793, 0.22183205361267255, 0.21943396764221745, 0.21580737042108922, 0.21056922427537472, 0.22237239074203075, 0.212072881622845, 0.19836540958911542, 0.1780824270538659, 0.14868200566931478, 0.11797663476669129, 0.09594391333588703, 0.07715372057155889, 0.06274130654361443, 0.05714791668830799, 0.06274130654365706, 0.07715372057161574, 0.09594391333588703, 0.11797663476667708, 0.14868200566931478, 0.1780824270538659, 0.19836540958841908, 0.212072881622845, 0.22237239074201653, 0.23091343202591474, 0.23737736077523408, 0.24222787361999565, 0.24575740357896336, 0.2481491165990377, 0.24908491977633673, 0.2481491165990377, 0.24575740357896336, 0.24222787362003828, 0.2373773607752412, 0.23091343202591474, 0.23533048963622605, 0.22341833236872333, 0.2090258538200871, 0.18454289853674055, 0.14648675448955828, 0.11797663476671261, 0.0965646225328527, 0.0782538069814791, 0.06662538303914545, 0.06662538303911703, 0.07825380698123752, 0.09656462253292375, 0.11797663476671261, 0.14648675448955828, 0.18454289853671213, 0.20902585382007288, 0.22341833236872333, 0.23533048963621184, 0.24645629091583743, 0.25604565034051063, 0.2638732773176926, 0.26973813329233565, 0.27401119238007254, 0.2761782139437159, 0.2761782139437372, 0.2740111923800441, 0.2697381332930391, 0.26387327731774946, 0.2560437420972903, 0.24645629091583032, 0.24255613160671885, 0.22853662554949494, 0.21233186398203685, 0.18454289853673345, 0.14868200566931478, 0.12174453412077924, 0.09968008144792861, 0.08305319680786738, 0.07673142302584068, 0.08305319680786738, 0.09968008144792861, 0.12174453412078634, 0.14868200566930057, 0.18454289853674055, 0.21233186398203685, 0.22853662554940968, 0.24255613160671885, 0.25639450235455996, 0.26952461106114356, 0.2812832166459742, 0.2910621250644141, 0.2984966838190388, 0.30295937593618305, 0.3043517018280042, 0.30295937593642464, 0.2984966838189962, 0.2910621250665457, 0.2812561927597841, 0.26952461106114356, 0.2563945023546381, 0.2448515249650356, 0.22853662554950205, 0.20902585382008, 0.1780824270538659, 0.150839690778227, 0.12804739193582293, 0.10802295303169274, 0.0963336603516467, 0.0963336603516467, 0.10802295303169274, 0.12804739193582293, 0.1508396907782128, 0.1780824270538659, 0.20902585382007288, 0.22853662554948073, 0.24485152496498586, 0.2611668393311035, 0.27741840435355414, 0.2928239257262675, 0.3066522734923396, 0.3181877397912416, 0.3263085075333123, 0.33015680568130534, 0.3301568056812414, 0.3263085075333194, 0.3181877397904458, 0.30664319254115924, 0.2928239257262817, 0.27741840435358256, 0.2611668393311035, 0.24255613160673306, 0.2234183323686949, 0.19836540958928595, 0.17061694797501303, 0.14968567222995688, 0.13146397985183, 0.11823345924230466, 0.11331183964553304, 0.11823345924230466, 0.13146397985184421, 0.1496856722299853, 0.17061694797521199, 0.19836540958828408, 0.2234183323686949, 0.24255613160671885, 0.2611668393311035, 0.279973056724387, 0.2984451507647563, 0.3158198253127793, 0.3311766121494486, 0.34318151446929335, 0.350310005488808, 0.35268288499335654, 0.35031000548877955, 0.34318151446927914, 0.3311766121494699, 0.31581982531142216, 0.29845062339137485, 0.27997305672484174, 0.2611668393310822, 0.23533048963622605, 0.2120728816228592, 0.18368435752066148, 0.16284388405492933, 0.14663063092551454, 0.1335170985559344, 0.1256259582917636, 0.1256259582917778, 0.1335170985559202, 0.14663063092553585, 0.16284388405492223, 0.18368435752064016, 0.212072881622845, 0.23533048963622605, 0.2563945023546381, 0.27741840435354703, 0.2984451507677903, 0.3188109893112312, 0.3374737327362425, 0.35293433027231913, 0.3633130873142605, 0.36816090908996557, 0.3681693649139106, 0.36331308731422496, 0.35294496236161166, 0.3374664592610941, 0.3188109893106059, 0.2984506233914459, 0.27741840435357545, 0.25639450235455286, 0.22237239074203075, 0.19338513562868087, 0.1697128415987379, 0.15479230638745634, 0.1430205577629593, 0.13377651899797627, 0.12999248242270767, 0.13377651899797627, 0.1430205577629593, 0.15479230638710817, 0.1697128415987379, 0.19338513562868798, 0.22237239074203075, 0.2464562909158161, 0.26952461106113645, 0.2928239257262817, 0.3158198253150317, 0.3374664592586072, 0.35610344527064797, 0.3694666319537703, 0.3768419601213395, 0.37915506589962433, 0.3768419601215811, 0.36946663195114837, 0.3561034452706551, 0.3374731530612394, 0.3158198253129285, 0.2928239257262817, 0.26952461106114356, 0.24645629091585164, 0.20295571167145, 0.17380584828498513, 0.15769763088273692, 0.14728481134286397, 0.13781003362568356, 0.13150759263150746, 0.13150759263150746, 0.13781003362571198, 0.14728481134285687, 0.1576976308827298, 0.17380584828497803, 0.2029557116714784, 0.23091343202591474, 0.25604565034055327, 0.2812832166455408, 0.3066522734923325, 0.3311766121494628, 0.3529378229744111, 0.3694666319582183, 0.3795699586983119, 0.3842341223084702, 0.38421655579884373, 0.3795699587031862, 0.36946663195582374, 0.35293782297470244, 0.331176612149477, 0.3066522734923396, 0.2812832166456758, 0.25604565034055327, 0.23091343202590764, 0.1797018853878143, 0.15834458933661466, 0.14743483944548075, 0.13834820487861776, 0.13037694682461165, 0.1270435582220557, 0.13037694682458323, 0.13834820487858224, 0.14743483944548075, 0.15834458933662177, 0.17970188538777876, 0.21056922427537472, 0.23737736077521276, 0.2638732773176571, 0.2910645412781463, 0.3181877397910853, 0.34318151446927914, 0.3633130873140118, 0.3768419601212898, 0.3842056278518058, 0.3865334761953534, 0.3842056278539445, 0.37684196012152427, 0.3633130873143102, 0.34318151446929335, 0.31818773979050263, 0.2910645412785726, 0.26387327731774235, 0.23737736077522698, 0.2105692242753605, 0.16081955557026362, 0.1459187100726922, 0.13608731348544723, 0.1270431862499919, 0.12093878634820499, 0.12093878634821209, 0.12704318624997057, 0.13608731348551828, 0.14591871007267798, 0.1608195555702423, 0.1877870028264681, 0.21580737042116027, 0.2422278736200525, 0.2697381332921367, 0.29849668381930883, 0.3263085075330707, 0.3503100054887369, 0.36816936491372587, 0.37911569858842853, 0.3842341223084631, 0.38420562785151446, 0.3791156985882509, 0.3681693649132285, 0.35031000548879376, 0.3263085075332697, 0.298514806184464, 0.26973813329156826, 0.24222787362002407, 0.2158073704211887, 0.18778700282645389, 0.14592595011598775, 0.13270331901448884, 0.12213485362466514, 0.11364112081101041, 0.1102494241801395, 0.11364112081096778, 0.12213485362437382, 0.13270331901448174, 0.14592595011600196, 0.16608585532769382, 0.19380920739000373, 0.21943396764222456, 0.24575740357897047, 0.27401119238006544, 0.302959375936112, 0.33015680568145456, 0.3526828849933068, 0.36815601617162486, 0.37684196012139637, 0.37956995870125354, 0.3768419601215669, 0.36816936491187846, 0.3526828849933068, 0.330156805681284, 0.3029593759361262, 0.27401119238007254, 0.24575740357896336, 0.21943396764223166, 0.1938092073899753, 0.1660858553276654, 0.13075899586556972, 0.11689842506948622, 0.10542699280701129, 0.0985535759678342, 0.0985535759678342, 0.1054269928070326, 0.11689842506950754, 0.13075899586557682, 0.14803652963334457, 0.1727832363595354, 0.19748976356095937, 0.22183205361267255, 0.2481491165990235, 0.2761782139437088, 0.3043517018277839, 0.3301568056813977, 0.35031000548875113, 0.3633130873142676, 0.3694666319579625, 0.36946663195402607, 0.3633130873142747, 0.3503100054888364, 0.3301568056813693, 0.30435170182845894, 0.2761782139437159, 0.2481491165990377, 0.2218320536127436, 0.19748976356095227, 0.17278323636118387, 0.14803652963333036, 0.11262352593976743, 0.097001190583768, 0.0854178570514108, 0.0812011583611536, 0.0854178570514108, 0.097001190583768, 0.11262352593976743, 0.12996798226795647, 0.1520827241580065, 0.17737775568241432, 0.19966471494916505, 0.22334916339341504, 0.24908491977631542, 0.27617821394380115, 0.3029593759366236, 0.32630850753324125, 0.34318151446927914, 0.35294496236149087, 0.35610344527063376, 0.3529266892192737, 0.34318151446928624, 0.3263085075331986, 0.3029593759362825, 0.2761782139437159, 0.2490849197762941, 0.22334916339339372, 0.19966471494916505, 0.17737775568241432, 0.1520827241580065, 0.12996798226792805, 0.08851254231704786, 0.07078901159886186, 0.060689274713794816, 0.060689274713794816, 0.07078901159886186, 0.08851254231707628, 0.10818940005012223, 0.12986956796500237, 0.15680287801056636, 0.17955776385476696, 0.20053351038428247, 0.2233491633933795, 0.2481491165990235, 0.27401119238007254, 0.2984966838195078, 0.31818773979040316, 0.331176612149477, 0.3374568306061221, 0.3374700402644564, 0.3311766121494628, 0.3181877397905808, 0.29849668381974226, 0.2740111923800441, 0.2481491165990377, 0.22334916339343636, 0.20053351038428957, 0.17955776385478828, 0.15680287801056636, 0.129869567965045, 0.10818940005011513, 0.06068821203136565, 0.0456118143712132, 0.04005475255581814, 0.04561181437117057, 0.06068821203162145, 0.08036626481417386, 0.10241054554465734, 0.13017196439993484, 0.15862805959559267, 0.17955776385478828, 0.19966471494916505, 0.2218320536125873, 0.24575740357897047, 0.269738133292563, 0.2910645412784305, 0.3066522734923325, 0.31581982531282904, 0.31881098931053486, 0.3158198253129001, 0.3066522734923183, 0.29106212506582096, 0.2697381332918667, 0.24575740357897757, 0.22183205361280045, 0.19966471494916505, 0.17955776385477407, 0.15862805959559978, 0.13017196439993484, 0.10241054554416706, 0.08036626481417386, 0.038158432534764586, 0.02686634910992325, 0.02686634910992325, 0.03815843253483564, 0.055965520644626565, 0.07604824987024017, 0.09954551522126565, 0.13017196439993484, 0.15680287801055925, 0.17737775568241432, 0.19748976356095227, 0.21943396764225298, 0.2422278736200667, 0.26387327731772814, 0.2812832166455621, 0.2928239257262817, 0.2984516331511742, 0.2984451507659145, 0.2928239257262675, 0.2812561927627115, 0.26387327731775656, 0.24222787362003828, 0.21943396764224588, 0.19748976356096648, 0.17737775568237168, 0.15680287801059478, 0.13017196439994194, 0.09954551522132249, 0.07604824987022596, 0.055965520644626565, 0.019436630015306378, 0.013315364007297603, 0.019436630015306378, 0.03496863398257943, 0.05455642334713673, 0.07604824987024017, 0.10241054554417417, 0.12986956796501659, 0.1520827241580207, 0.17278323635773063, 0.1938092073899611, 0.2158073704211887, 0.23737736077522698, 0.25604565034051063, 0.26952461106114356, 0.27741840435356835, 0.2799730567250549, 0.27741840435355414, 0.26952461106114356, 0.25604565034053905, 0.23737736077521987, 0.21580737042112474, 0.1938092073899682, 0.17278323636047332, 0.1520827241580065, 0.1298695679650237, 0.10241054554415996, 0.07604824987025438, 0.05455642334713673, 0.03496863398257233, 0.005541623615314961, 0.005541623615314961, 0.016933037985893407, 0.03496863398256522, 0.05596552064464788, 0.08036626481413833, 0.10818940005012223, 0.12996798226794226, 0.14803652963331615, 0.1660858553276796, 0.187787002826461, 0.21056922427537472, 0.23091343202591474, 0.24645629091584453, 0.2563945023545955, 0.2611668393310964, 0.2611668393310964, 0.2563945023546097, 0.24645629091583032, 0.23091343202594317, 0.21056922427537472, 0.1877870028264681, 0.16608585532770803, 0.14803652963334457, 0.12996798226791384, 0.10818940005012223, 0.08036626481415965, 0.055965520644612354, 0.03496863398257233, 0.016933037985893407])
gamma = am.defect.GammaSurface(a1vect=a1vect, a2vect=a2vect, a1=a1, a2=a2,
E_gsf=E_gsf, box=box, delta=delta)
3.2. Class attributes
The GammaSurface class has the following attributes for the data
a1vect, a2vect the two shifting vectors as given.
box the unit cell box as given.
planenormal a Cartesian vector normal to the fault plane.
data a pandas.DataFrame tabulating the measured stacking fault data. The data columns are
a1 fractional coordinates along the a1vect.
a2 fractional coordinates along the a2vect.
E_gsf generalized stacking fault energies measured for each (a1, a2) fault shift.
delta (optional) relaxation distances measured for each (a1, a2) fault shift.
[3]:
print('gamma.a1vect ->', gamma.a1vect)
print('gamma.a2vect ->', gamma.a2vect)
print('gamma.planenormal ->', gamma.planenormal)
print('gamma.box ->')
print(gamma.box)
gamma.a1vect -> [ 0. -0.5 0.5]
gamma.a2vect -> [ 0.5 -0.5 0. ]
gamma.planenormal -> [0.57735027 0.57735027 0.57735027]
gamma.box ->
avect = [ 4.045, 0.000, 0.000]
bvect = [ 0.000, 4.045, 0.000]
cvect = [ 0.000, 0.000, 4.045]
origin = [ 0.000, 0.000, 0.000]
[4]:
gamma.data
[4]:
a1 | a2 | E_gsf | delta | |
---|---|---|---|---|
0 | 0.000000 | 0.000000 | 0.000000 | 0.000000 |
1 | 0.033333 | 0.000000 | 0.000328 | 0.005542 |
2 | 0.066667 | 0.000000 | 0.001320 | 0.019437 |
3 | 0.100000 | 0.000000 | 0.003008 | 0.038158 |
4 | 0.133333 | 0.000000 | 0.005299 | 0.060688 |
... | ... | ... | ... | ... |
895 | 0.833333 | 0.966667 | 0.009792 | 0.108189 |
896 | 0.866667 | 0.966667 | 0.007090 | 0.080366 |
897 | 0.900000 | 0.966667 | 0.004494 | 0.055966 |
898 | 0.933333 | 0.966667 | 0.002400 | 0.034969 |
899 | 0.966667 | 0.966667 | 0.001009 | 0.016933 |
900 rows × 4 columns
3.3. Saving and loading data model
The GammaSurface.model() method allows for the saving and loading of a GammaSurface object’s data as a JSON/XML data model.
Parameters
model (str, file-like object or DataModelDict, optional) XML/JSON content to extract gamma surface energy from. If not given, model content will be generated.
length_unit (str, optional) Units to report delta displacement values in when a new model is generated. Default value is ‘angstrom’.
energyperarea_unit (str, optional) Units to report fault energy values in when a new model is generated. Default value is ‘mJ/m^2’.
Returns
(DataModelDict) A data model containing the stacking fault data of the GammaSurface object. Returned if model is not given.
3.3.1. Save data model
Calling GammaSurface.model() without supplying the ‘model’ parameter will return a data model of the content.
[5]:
model = gamma.model()
with open('gamma.json', 'w') as f:
model.json(fp=f)
3.3.2. Load data model
The saved data can then be loaded by using the ‘model’ parameter when initializing a GammaSurface, or with the GammaSurface.model() method.
[6]:
gamma = am.defect.GammaSurface(model='gamma.json')
4. Plotting methods
4.1. E_gsf_line_plot()
Generates a line plot for the interpolated generalized stacking fault energy along a specified vector in the (a1, a2) plane.
Parameters
vect (numpy.array, optional) Vector to plot the gsf along. If box is set, this vect will be a lattice vector, otherwise it will be a Cartesian vector. Must be in the plane defined by the GammaSurface object’s a1vect and a2vect vectors. Default value will use the set a1vect.
num (int, optional) The number of points to evaluate the generalized stacking fault energy for. Default value is 100 if smooth is True, otherwise is number of unique a1 values from 0 to 1.
smooth (bool, optional) If True (default), then plot shows smooth interpolated values. If False, plot shows nearest raw data values.
length_unit (str, optional) The unit of length to display the x-axis coordinates in. Default value is ‘Å’.
energyperarea_unit (str, optional) The unit of energy per area to display the stacking fault energies in. Default value is ‘eV/Å^2’.
figsize (tuple, optional) The x,y size of the figure to return. Default value is (10, 6).
fig (matplotlib.figure, optional) An existing figure object to add the new plot to. If not given, a new figure is generated.
**kwargs (dict, optional) Additional keywords are passed into the underlying matplotlib.pyplot.plot(). This allows control of such things like line color, style, etc.
Returns
(matplotlib.figure)
If vect is not given, will use a1vect
[7]:
# Generate RBF interpolated plot
fig = gamma.E_gsf_line_plot(fmt='k-', label='RBF')
# Generate measured (nearest) plot and append to above
fig = gamma.E_gsf_line_plot(smooth=False, fmt='k:o', fig=fig, label='measured')
# Additional plot manipulations
plt.legend(loc=2, fontsize='x-large')
plt.show()
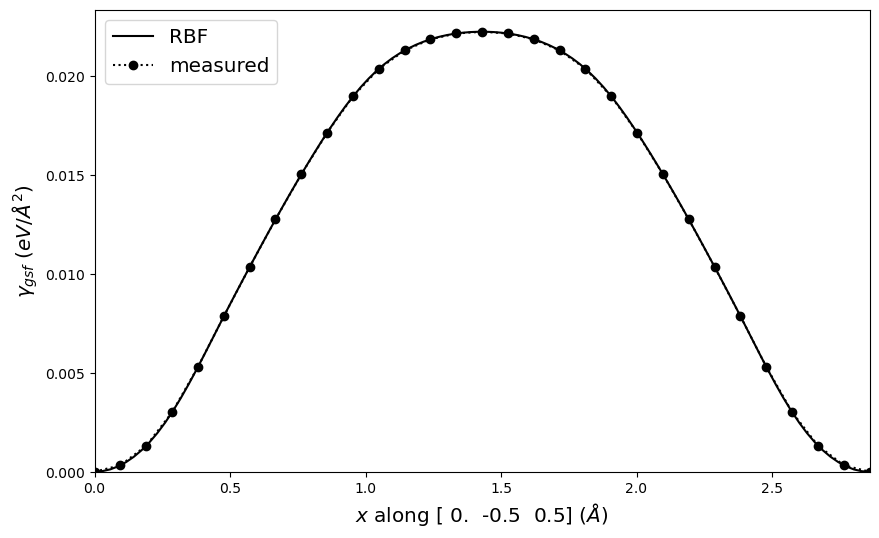
The important direction for fcc {111} stacking faults is a/2<112>, and energy values in mJ/m^2
[8]:
# Generate RBF interpolated plot
vect = np.array([1, 1, -2]) / 2
fig = gamma.E_gsf_line_plot(vect=vect, fmt='k-', energyperarea_unit='mJ/m^2', label='RBF')
# Generate measured (nearest) plot and append to above
fig = gamma.E_gsf_line_plot(vect=vect, smooth=False, fmt='k:o',
energyperarea_unit='mJ/m^2', fig=fig, label='measured')
# Additional plot manipulations
plt.legend(loc=2, fontsize='x-large')
plt.show()
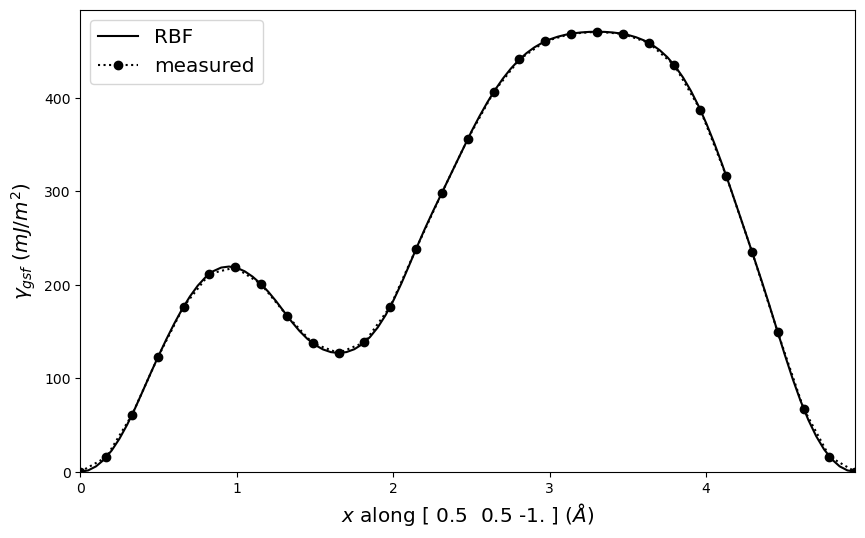
4.2. delta_line_plot()
Generates a line plot for the interpolated relaxation distances along a specified vector in the (a1, a2) plane.
Parameters
vect (numpy.array, optional) Vector to plot the gsf along. If box is set, this vect will be a lattice vector, otherwise it will be a Cartesian vector. Must be in the plane defined by the GammaSurface object’s a1vect and a2vect vectors. Default value will use the set a1vect.
num (int, optional) The number of points to evaluate the generalized stacking fault energy for. Default value is 100 if smooth is True, otherwise is number of unique a1 values from 0 to 1.
smooth (bool, optional) If True (default), then plot shows smooth interpolated values. If False, plot shows nearest raw data values.
length_unit (str, optional) The unit of length to display the x-axis coordinates in. Default value is ‘Å’.
figsize (tuple, optional) The x,y size of the figure to return. Default value is (10, 6).
fig (matplotlib.figure, optional) An existing figure object to add the new plot to. If not given, a new figure is generated.
**kwargs (dict, optional) Additional keywords are passed into the underlying matplotlib.pyplot.plot(). This allows control of such things like line color, style, etc.
Returns
(matplotlib.figure)
If vect is not given, will use a1vect
[9]:
# Generate RBF interpolated plot
fig = gamma.delta_line_plot(fmt='k-', label='RBF')
# Generate measured (nearest) plot and append to above
fig = gamma.delta_line_plot(smooth=False, fmt='k:o', fig=fig, label='measured')
# Additional plot manipulations
plt.legend(loc=2, fontsize='x-large')
plt.show()
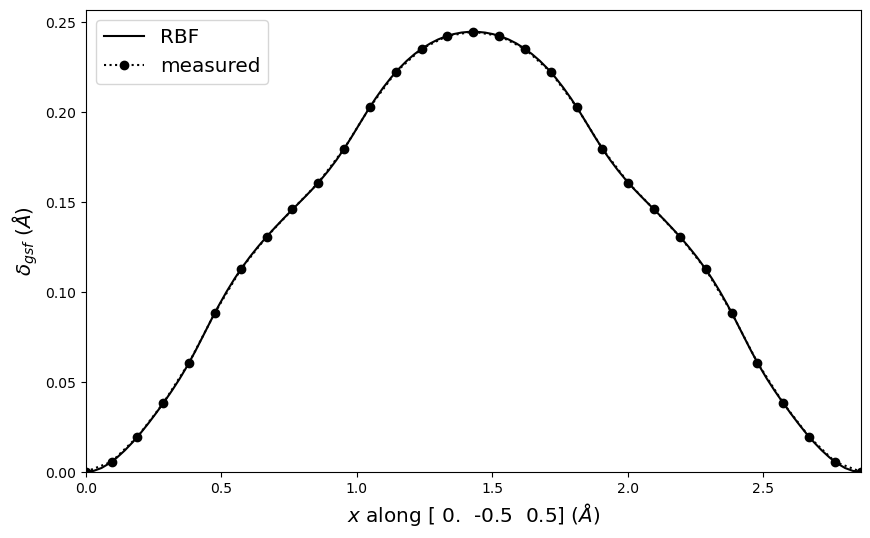
The important direction for fcc {111} stacking faults is a/2<112>
[10]:
# Generate RBF interpolated plot
vect = np.array([1, 1, -2]) / 2
fig = gamma.delta_line_plot(vect=vect, fmt='k-', label='RBF')
# Generate measured (nearest) plot and append to above
fig = gamma.delta_line_plot(vect=vect, smooth=False, fmt='k:o', fig=fig, label='measured')
# Additional plot manipulations
plt.legend(loc=2, fontsize='x-large')
plt.show()
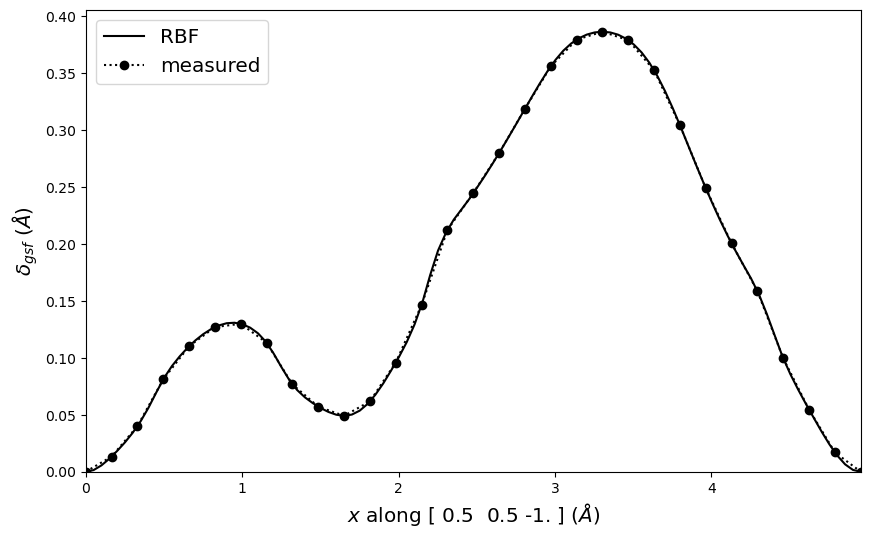
4.3. E_gsf_surface_plot()
Creates a 2D surface plot from the stacking fault energy values.
Parameters
normalize (bool, optional) Flag indicating if axes are Cartesian (False, default) or normalized by a1, a2 vectors (True).
smooth (bool, optional) If True (default), then plot shows smooth interpolated values. If False, plot shows nearest raw data values.
a1vect (np.array, optional) Crystal vector for the a1 vector to use for plotting. Default value of None uses the saved a1vect.
a2vect (np.array, optional) Crystal vector for the a2 vector to use for plotting. Default value of None uses the saved a2vect.
xvect (numpy.array, optional) Crystal vector to align with the plotting x-axis for non-normalized plots. If not given, this is taken as the Cartesian of a1vect.
length_unit (str, optional) The unit of length to display non-normalized axes values in. Default value is ‘Å’.
energyperarea_unit (str, optional) The unit of energy per area to display the stacking fault energies in. Default value is ‘eV/Å^2’.
numx (int, optional) The number of plotting points to use along the x-axis. Default value is 100.
numy (int, optional) The number of plotting points to use along the y-axis. Default value is 100.
figsize (tuple or None, optional) The figure’s x,y dimensions. If None (default), the values are scaled such that the x,y spacings are approximately equal, and the larger of the two values is set to 10.
**kwargs (dict, optional) Additional keywords are passed into the underlying matplotlib.pyplot.pcolormesh(). This allows control of such things like the colormap (cmap).
Returns
(matplotlib.figure)
Default setting
[11]:
fig = gamma.E_gsf_surface_plot()
plt.show()
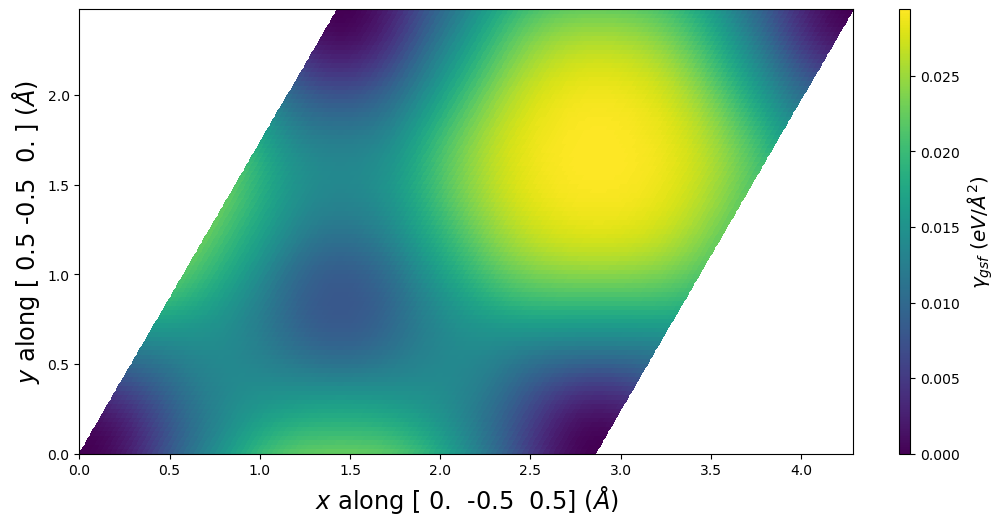
Plotting actual measured values
[12]:
fig = gamma.E_gsf_surface_plot(smooth=False, normalize=True, cmap='gray')
plt.show()
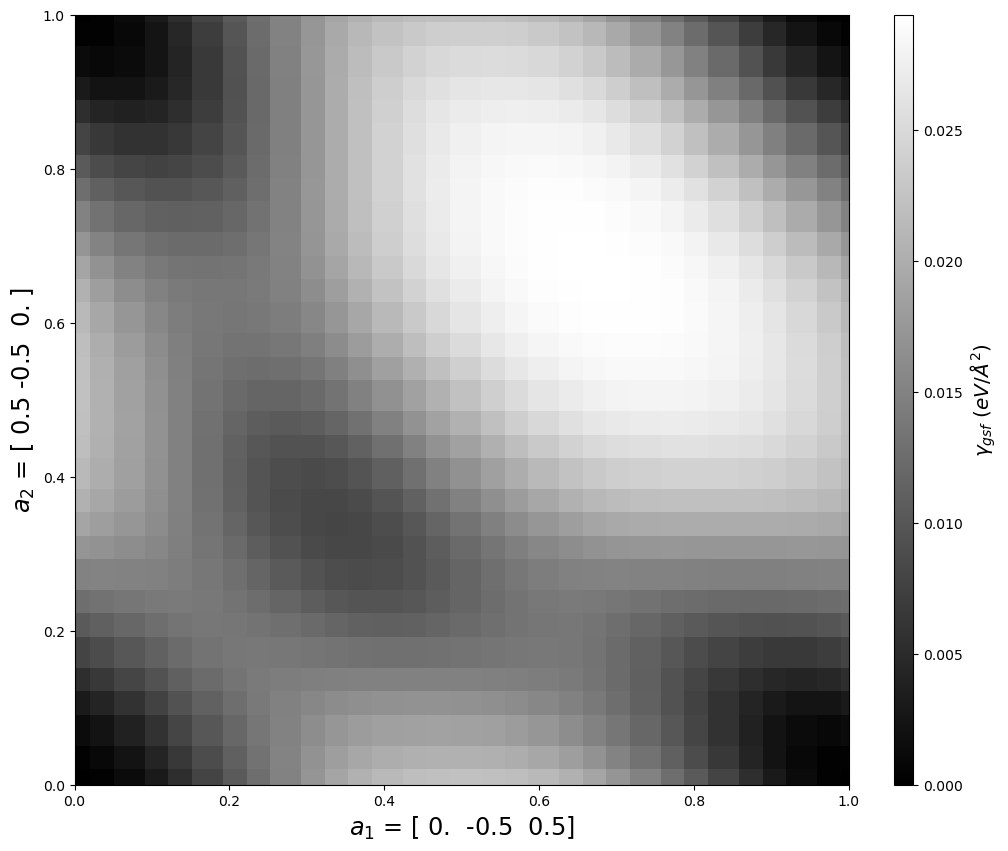
Specifying a different a1vect and a2vect to use for plotting
[13]:
plot_a1vect = np.array([1, 1, -2]) / 2
plot_a2vect = np.array([1,-1, 0]) / 2
fig = gamma.E_gsf_surface_plot(a1vect=plot_a1vect, a2vect=plot_a2vect, energyperarea_unit='mJ/m^2', cmap='jet')
plt.show()
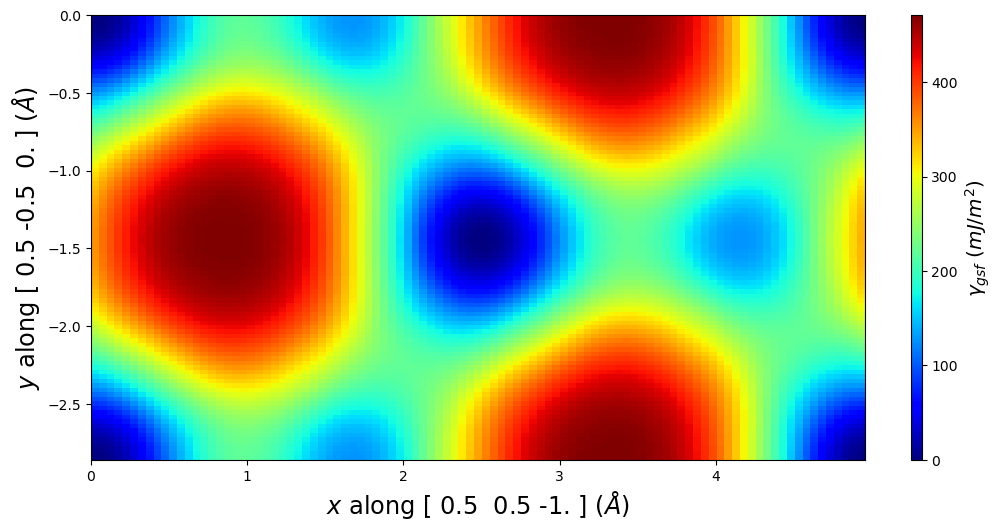
4.4. delta_surface_plot()
Creates a 2D surface plot from the relaxation distance values.
Parameters
normalize (bool, optional) Flag indicating if axes are Cartesian (False, default) or normalized by a1, a2 vectors (True).
smooth (bool, optional) If True (default), then plot shows smooth interpolated values. If False, plot shows nearest raw data values.
a1vect (np.array, optional) Crystal vector for the a1 vector to use for plotting. Default value of None uses the saved a1vect.
a2vect (np.array, optional) Crystal vector for the a2 vector to use for plotting. Default value of None uses the saved a2vect.
xvect (numpy.array, optional) Crystal vector to align with the plotting x-axis for non-normalized plots. If not given, this is taken as the Cartesian of a1vect.
length_unit (str, optional) The unit of length to display non-normalized axes values in. Default value is ‘Å’.
numx (int, optional) The number of plotting points to use along the x-axis. Default value is 100.
numy (int, optional) The number of plotting points to use along the y-axis. Default value is 100.
figsize (tuple or None, optional) The figure’s x,y dimensions. If None (default), the values are scaled such that the x,y spacings are approximately equal, and the larger of the two values is set to 10.
**kwargs (dict, optional) Additional keywords are passed into the underlying matplotlib.pyplot.pcolormesh(). This allows control of such things like the colormap (cmap).
Returns
(matplotlib.figure)
Default setting
[14]:
fig = gamma.delta_surface_plot()
plt.show()
/home/lmh1/Python-packages/atomman/atomman/defect/GammaSurface.py:1145: UserWarning: The input coordinates to pcolormesh are interpreted as cell centers, but are not monotonically increasing or decreasing. This may lead to incorrectly calculated cell edges, in which case, please supply explicit cell edges to pcolormesh.
plt.pcolormesh(x_grid, y_grid, C, **kwargs)
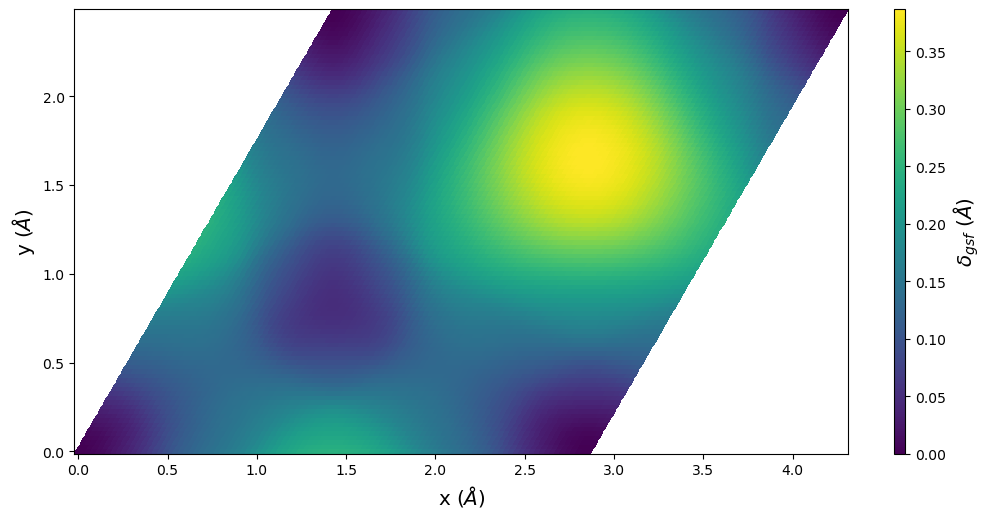
Plotting actual measured values
[15]:
fig = gamma.delta_surface_plot(smooth=False, normalize=True, cmap='gray')
plt.show()
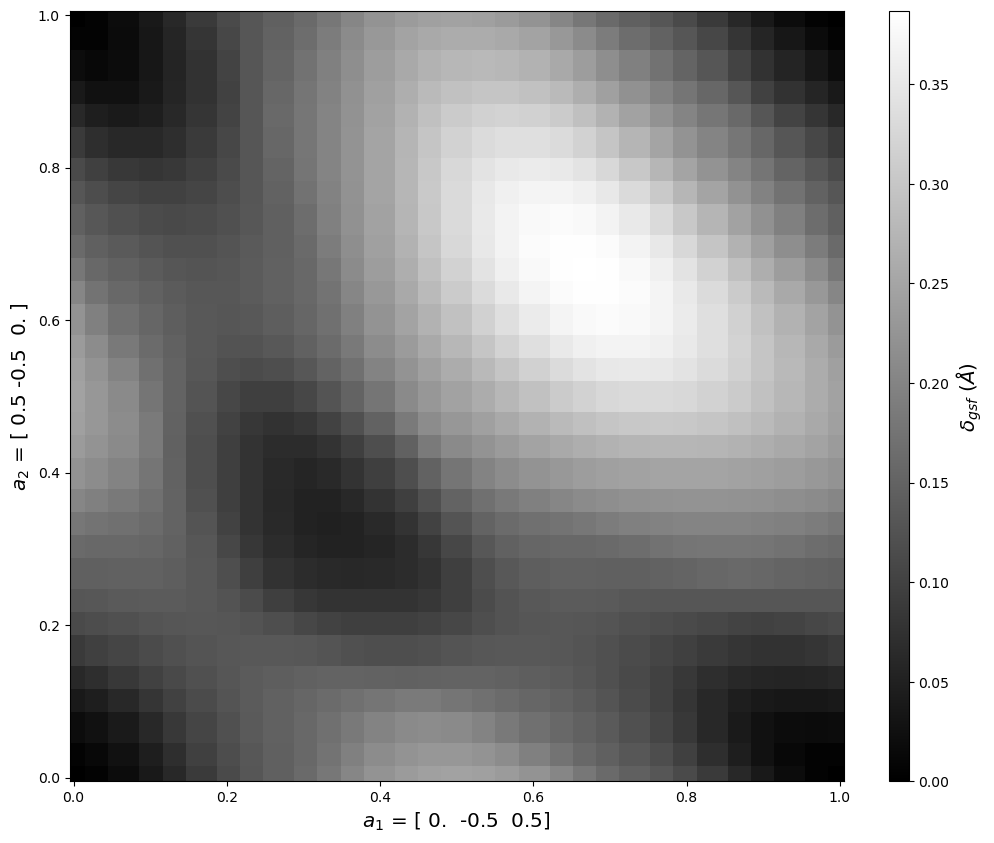
Specifying a different a1vect and a2vect to use for plotting
[16]:
plot_a1vect = np.array([1, 1, -2]) / 2
plot_a2vect = np.array([1,-1, 0]) / 2
fig = gamma.delta_surface_plot(a1vect=plot_a1vect, a2vect=plot_a2vect, cmap='jet')
plt.show()
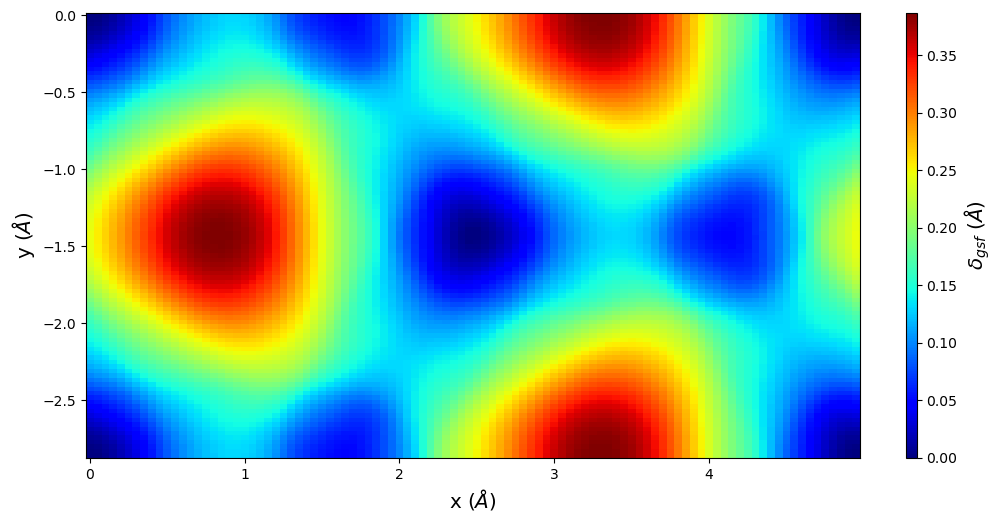
5. Minimum energy slip path
Added version 1.3.7
The path() and build_path() methods allow for the construction of atomman.mep.Path objects where the energy function associated with the path coordinates is automatically set using the generalized stacking fault energy. path() allows for all 2D coordinates along the path to be specified, while build_path() allows for a path to be constructed based only on the endpoints and optionally one intermediate junction point.
Documentation coming soon…
6. Utility methods
The remaining class methods are utility methods for generating the plots. Many are focused on transforming between different coordinate systems:
(a1, a2) : fractional coordinates along a1vect and a2vect.
pos : Cartesian position coordinates.
(x, y) : plotting coordinates.
Transforming from (a1, a2) to pos requires a1vect, a2vect, and optionally the box. Transforming from pos to (x, y) requires specifying which Cartesian vector to align with the x-axis of the plot.
6.1. E_gsf()
Returns values for generalized stacking fault energy interpolated from the raw data. Values can be obtained relative to a1, a2 fractional coordinates, x, y plotting coordinates, or pos Cartesian coordinates.
Parameters
a1 (float(s), optional) Fractional coordinate(s) along a1vect.
a2 (float(s), optional) Fractional coordinate(s) along a2vect.
pos (np.array, optional) 3D Cartesian position vector(s).
x (float(s), optional) Plotting x coordinate(s).
y (float(s), optional) Plotting y coordinate(s).
a1vect (np.array, optional) Vector for the a1 fractional coordinates. Default value of None uses the saved a1vect.
a2vect (np.array, optional) Vector for the a2 fractional coordinates. Default value of None uses the saved a2vect.
xvect (np.array, optional) Cartesian vector corresponding to the plotting x-axis. If None (default), this is taken as the Cartesian of a1vect.
smooth (bool, optional) If True (default) the returned values are smoothed using a RBF fit. If False, the closest measured values are returned.
Returns
(float or numpy.ndarray) the energy values.
[17]:
print(f'Close to E_isf = {uc.get_in_units(gamma.E_gsf(a1=1/3, a2=1/3), "mJ/m^2")} mJ/m^2')
print(f'Close to E_usf = {uc.get_in_units(gamma.E_gsf(a1=2/3, a2=2/3), "mJ/m^2")} mJ/m^2')
Close to E_isf = 127.08436673732754 mJ/m^2
Close to E_usf = 471.02588197516434 mJ/m^2
6.2 delta()
Returns values for the relaxation distance interpolated from the raw data. Values can be obtained relative to a1, a2 fractional coordinates, x, y plotting coordinates, or pos Cartesian coordinates.
Parameters
a1 (float(s), optional) Fractional coordinate(s) along a1vect.
a2 (float(s), optional) Fractional coordinate(s) along a2vect.
pos (np.array, optional) 3D Cartesian position vector(s).
x (float(s), optional) Plotting x coordinate(s).
y (float(s), optional) Plotting y coordinate(s).
a1vect (np.array, optional) Vector for the a1 fractional coordinates. Default value of None uses the saved a1vect.
a2vect (np.array, optional) Vector for the a2 fractional coordinates. Default value of None uses the saved a2vect.
xvect (np.array, optional) Cartesian vector corresponding to the plotting x-axis. If None (default), this is taken as the Cartesian of a1vect.
smooth (bool, optional) If True (default) the returned values are smoothed using a RBF fit. If False, the closest measured values are returned.
Returns
(float or numpy.ndarray) the relaxation distance values.
[18]:
print(f'Close to δ_isf = {uc.get_in_units(gamma.delta(a1=1/3, a2=1/3), "angstrom")} angstrom')
print(f'Close to δ_usf = {uc.get_in_units(gamma.delta(a1=2/3, a2=2/3), "angstrom")} angstrom')
Close to δ_isf = 0.049080885280148756 angstrom
Close to δ_usf = 0.38653347619535694 angstrom
6.3 Conversion methods
Parameters as defined above
a12_to_pos(a1, a2, a1vect=None, a2vect=None)
pos_to_xy(pos, xvect=None)
a12_to_xy(a1, a2, a1vect=None, a2vect=None, xvect=None)
pos_to_a12(pos, a1vect=None, a2vect=None)
xy_to_pos(x, y, xvect=None)
xy_to_a12(x, y, a1vect=None, a2vect=None, xvect=None)
File cleanup
[19]:
Path('gamma.json').unlink()