examples.diffusion.electrostaticsΒΆ
Solve the Poisson equation in one dimension.
The Poisson equation is a particular example of the steady-state diffusion equation. We examine a few cases in one dimension.
>>> from fipy import CellVariable, Grid1D, Viewer, DiffusionTerm
>>> nx = 200
>>> dx = 0.01
>>> L = nx * dx
>>> mesh = Grid1D(dx = dx, nx = nx)
Given the electrostatic potential ,
>>> potential = CellVariable(mesh=mesh, name='potential', value=0.)
the permittivity ,
>>> permittivity = 1
the concentration of the
component with valence
(we consider only a single component
with
valence with
)
>>> electrons = CellVariable(mesh=mesh, name='e-')
>>> electrons.valence = -1
and the charge density ,
>>> charge = electrons * electrons.valence
>>> charge.name = "charge"
The dimensionless Poisson equation is
>>> potential.equation = (DiffusionTerm(coeff = permittivity)
... + charge == 0)
Because this equation admits an infinite number of potential profiles, we must constrain the solution by fixing the potential at one point:
>>> potential.constrain(0., mesh.facesLeft)
First, we obtain a uniform charge distribution by setting a uniform concentration
of electrons .
>>> electrons.setValue(1.)
and we solve for the electrostatic potential
>>> potential.equation.solve(var=potential)
This problem has the analytical solution
>>> x = mesh.cellCenters[0]
>>> analytical = CellVariable(mesh=mesh, name="analytical solution",
... value=(x**2)/2 - 2*x)
which has been satisfactorily obtained
>>> print(potential.allclose(analytical, rtol = 2e-5, atol = 2e-5))
1
If we are running the example interactively, we view the result
>>> from fipy import input
>>> if __name__ == '__main__':
... viewer = Viewer(vars=(charge, potential, analytical))
... viewer.plot()
... input("Press any key to continue...")
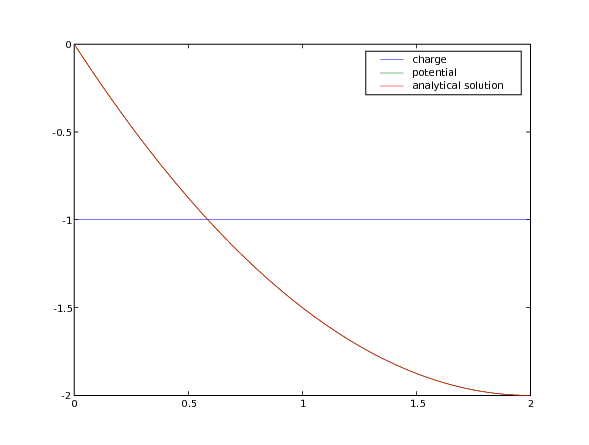
Next, we segregate all of the electrons to right side of the domain
>>> x = mesh.cellCenters[0]
>>> electrons.setValue(0.)
>>> electrons.setValue(1., where=x > L / 2.)
and again solve for the electrostatic potential
>>> potential.equation.solve(var=potential)
which now has the analytical solution
>>> analytical.setValue(-x)
>>> analytical.setValue(((x-1)**2)/2 - x, where=x > L/2)
>>> print(potential.allclose(analytical, rtol = 2e-5, atol = 2e-5))
1
and again view the result
>>> from fipy import input
>>> if __name__ == '__main__':
... viewer.plot()
... input("Press any key to continue...")
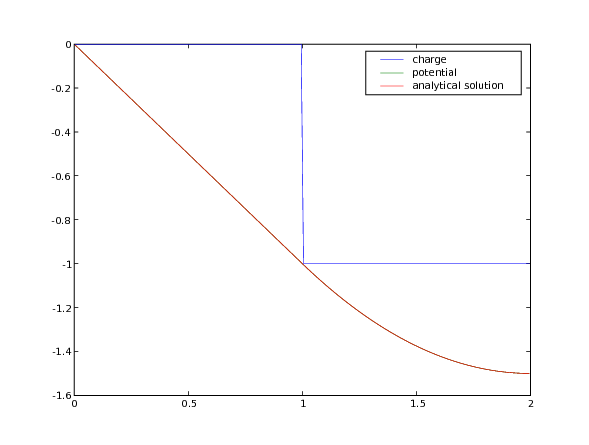
Finally, we segregate all of the electrons to the left side of the domain
>>> electrons.setValue(1.)
>>> electrons.setValue(0., where=x > L / 2.)
and again solve for the electrostatic potential
>>> potential.equation.solve(var=potential)
which has the analytical solution
We again verify that the correct equilibrium is attained
>>> analytical.setValue((x**2)/2 - x)
>>> analytical.setValue(-0.5, where=x > L / 2)
>>> print(potential.allclose(analytical, rtol = 2e-5, atol = 2e-5))
1
and once again view the result
>>> if __name__ == '__main__':
... viewer.plot()
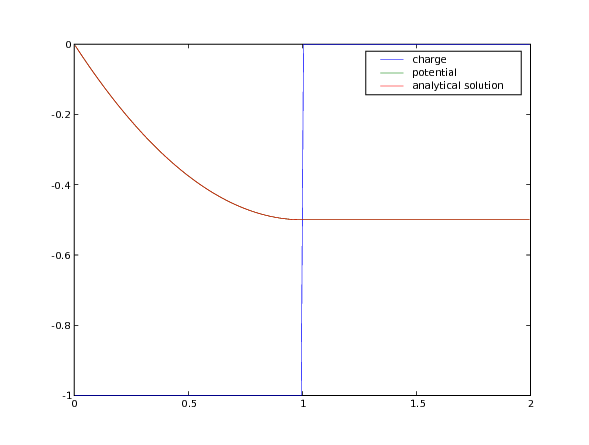